Project: Luigi's Pizzeria - Adding CSS to the Homepage
Requirements
- Each page will have a navigation bar with links to each of the other pages.
- The navigation bar will be responsive:
- Screens smaller than
768px
will display the links vertically. - Screens
768px
or larger will display the links horizontally. - The links will start off orange but when hovered over, the links should turn black.
- Screens smaller than
- The navigation bar will have a light grey background.
- The font for the text on all the pages will be something different from the default font, perhaps something "Gothic".
- The copyright on each page will have a dark grey background and the text color will be white and also centered at the bottom of the page.
- The main content of the homepage will be centered in the middle of the page.
- The homepage will have a background image for the main content that gives a parallax effect.
Instructions
- Create a new subfolder in the pizzeria project and call it "css".
- In the subfolder, create a new file called "styles.css"
- In the "index.html" file, add a "link" to the "styles.css" file after the title tags:
<!-- Link to styles.css --> <link rel="stylesheet" href="./css/styles.css">
- Save.
- If "Live Server" is not already running, right-click anywhere in the editor and select "Open with Live Server" from the context menu.
- Move the h1 and unordered list elements inside a new div tag that has a class of "navbar" so that it looks like this:
<!-- Wrap Navigation List in a DIV --> <div class="navbar"> <h1>Luigi's Pizzeria</h1> <ul> <li><a href="./index.html">Home</a></li> <li><a href="./pizzas.html">Our Pizzas</a></li> <li><a href="./prices.html">Prices</a></li> <li><a href="./contact.html">Contact</a></li> </ul> </div>
- Save.
- Remove the background image by commenting out the following lines of the HTML (we will be re-adding it via CSS later):
<!-- <img src="./images/background.jpg" alt="background image">
<br> -->
- Save.
- Create a new div with a class of "main" under the commented out img tag:
<div class="main"> </div>
- Save.
- Inside the div with a class of "main", create another div with a class of "main-content":
<div class="main-content"> </div>
- Save.
- Create a new h2 header by adding the following code inside the div with the class of "main-content":
<h2>Welcome to Luigi's Pizzeria</h2>
- Save.
- Move the paragraph with the restaurant description inside the div with the class of "main-content" under the h2 heading so that the code looks like this:
<!-- Main Content--> <div class="main"> <div class="main-content"> <h2>Welcome to Luigi's Pizzeria</h2> <p> Luigi's Pizzeria is a warm and inviting restaurant specializing in delicious, authentic Italian <a href="https://en.wikipedia.org/wiki/Pizza" target="_blank">pizza</a>. With its charming decor and friendly atmosphere, Luigi's is the perfect place to enjoy a meal with family and friends. The menu features a variety of tasty <a href="https://en.wikipedia.org/wiki/Pizza" target="_blank">pizza</a> options, made with fresh ingredients and cooked to perfection in a wood-fired oven. Whether you're in the mood for a classic cheese, a spicy pepperoni, or a unique specialty pie, Luigi's has something for everyone. And with competitive prices, you can enjoy a delicious meal without breaking the bank. Visit Luigi's Pizzeria today and taste the difference for yourself! </p> </div> </div>
- Save.
- Add a div with a class of "footer" after the opening and closing tags of the div with class of "main":
<!-- Footer --> <div class="footer"> </div>
- Save.
- Move the copyright paragraph inside the div with class of "footer" so that it looks like the following:
<!-- Footer --> <div class="footer"> <p>© 2023 All rights reserved.</p> </div>
- Save.
- In the "styles.css" file, add the following to setup the variables that we will be using throughout css:
/* Variables */ :root { --primaryColor: #f15015; --primaryBlack: #222; --primaryWhite: #fff; --primarySpacing: 0.2rem; --darkGrey: #afafaf; }
- Save.
- In the "styles.css" file, add the following to reset the default browser settings:
* { margin: 0; padding: 0; box-sizing: border-box; }
- Save.
- In the "styles.css" file, add the following to set the font family, color, background, and line-height for the all pages using this "css" file:
body { font-family: 'Franklin Gothic Medium', 'Arial Narrow', Arial, sans-serif; color: var(--primaryBlack); background: var(--primaryWhite); line-height: 1.5; }
- Save.
- In the "styles.css" file, add the following to set the font color and text decoration (remove the underline) of all links for the all pages using this "css" file:
a { text-decoration: none; color: var(--primaryColor); }
- Save.
- In the "styles.css" file, add the following to set width and display type of all images for the all pages using this "css" file:
img { width: 100%; display: block; }
- Save.
- In the "styles.css" file, add the following to set the text transform, letter spacing, and bottom margin of all h1 and h2 elements for the all pages using this "css" file:
h1, h2 { text-transform: capitalize; letter-spacing: var(--primarySpacing); } h1 { font-size: 3rem; margin-bottom: 1.25rem; } h2 { margin-bottom: 0; }
- Save.
- In the "styles.css" file, add the following to set the font weight, font size, and line height of all p elements for the all pages using this "css" file:
p { font-weight: 300; font-size: 0.7rem; line-height: 2; }
- Save.
- In the "styles.css" file, add the following to set the background color and padding of the navigation bar for the all pages using this "css" file:
.navbar { background: var(--darkGrey); padding: 0 2rem; }
- Save.
- In the "styles.css" file, add the following to remove the bullet points from the list items used in the navigation bar for the all pages using this "css" file:
.navbar li { list-style: none; }
- Save.
- In the "styles.css" file, add the following to format the navigation items so that they display vertically by default for the all pages using this "css" file:
.navbar a { text-transform: capitalize; display: block; padding: 1rem 0; letter-spacing: var(--primarySpacing); }
- Save.
- In the "styles.css" file, add the following to make it so that the links change color when you hover of it for the all pages using this "css" file:
.navbar a:hover { color: var(--primaryBlack); }
- Save.
- In the "styles.css" file, add the following media query to make it so that the navigation bar displays all links horizontally when the screen size is 768 pixels or greater for the all pages using this "css" file:
@media screen and (min-width: 768px) { .navbar ul { max-width: 1170px; margin: 0 auto; display: grid; grid-template-columns: repeat(4, 1fr); } .navbar a { text-align: center; } }
- Save.
- In the "styles.css" file, add the following to format the "main" area so that there is a background image overlay and content centered for all pages using this "css" file:
/* main */ .main { min-height: 90vh; background: linear-gradient(rgba(0, 0, 0, 0.75), rgba(0, 0, 0, 0.75)), url("../images/background.jpg") center/cover fixed no-repeat; color: var(--primaryWhite); display: grid; justify-content: center; align-items: center; } .main-content { padding: 0 1rem; } .main-content p { width: 80%; max-width: 560px; font-size: 1rem; }
- Save.
- In the "styles.css" file, add the following to format the "footer" area for all pages using this "css" file:
/* footer */ .footer { background: var(--primaryBlack); color: var(--primaryWhite); text-transform: capitalize; text-align: center; padding: 0.5rem 0; } .footer p { font-size: 1.2rem; }
- Save.
- In the browser, you should see the following:
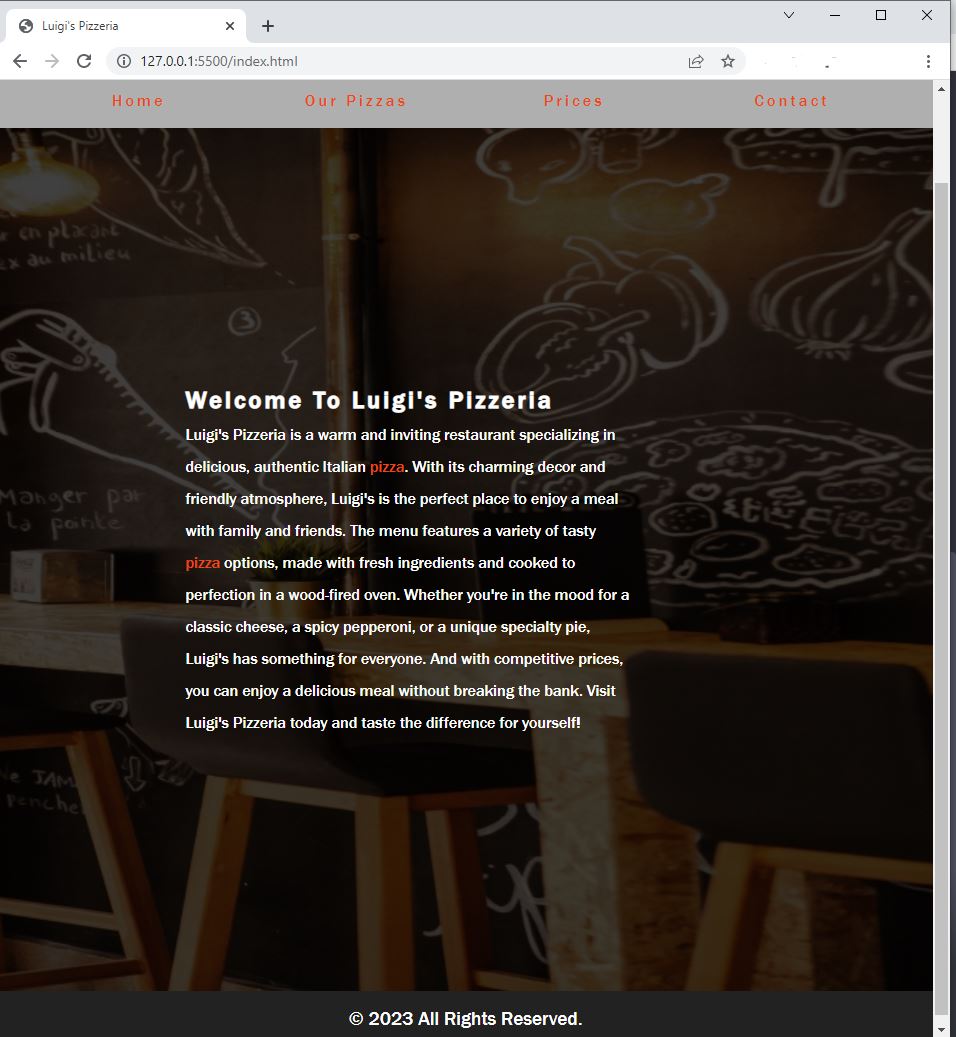
Code Walkthrough and Explanation
For the homepage, all requirements were met. The navigation bar is dynamic and will look slightly different when the browser is resized. The content is nicely centered in the main area with a background image overlay which when you scroll will give a parallax effect. The footer is clear and centered at the bottom of the page. This was a longer setup but since we will be reusing the "style.css" file for the other pages, we will only need to add additional styling for the other pages. The navigation bar, footer, and generic settings for the main content will automatically apply to the other pages once we've applied the appropriate classes and ids to the HTML.
Video and Code References
Questions? Subscribe and ask in the video comments: